MCQ Practice Questions
59 important questions on MCQ Practice Questions
Which of the following stands true about default modifier of class members?
A - By default, variables, methods and constructors can be accessed by subclass only.
B - By default, variables, methods and constructors can be accessed by any class lying in any package.
C - By default, variables, methods and constructors can be accessed by any class lying in the same package.
D - None of the above.
Can a top level class be private or protected?
A - True.
B - False.
--> No, a top level class can not be private or protected. It can have either "public" or no modifier.
Which data type value is returned by all transcendental math functions?
a) int
b) float
c) double
d) long
- Higher grades + faster learning
- Never study anything twice
- 100% sure, 100% understanding
What will this output be?
p.p1 {margin: 0.0px 0.0px 0.0px 0.0px; font: 12.0px Monaco} span.s1 {color: #931a68} span.s2 {color: #7e504f} span.s3 {color: #0326cc} span.Apple-tab-span {white-space:pre} public static void main(String[] args) {
int g = 3;
System.out.print(++g * 8);
Which of these coding types is used for data type characters in Java?
a) ASCII
b) ISO-LATIN-1
c) UNICODE
d) None of the mentioned
--> Explanation: Unicode defines fully international character set that can represent all the characters found in all human languages. Its range is from 0 to 65536.
Which one is a valid declaration of a boolean?
a) boolean b1 = 1;
b) boolean b2 = ‘false’;
c) boolean b3 = false;
d) boolean b4 = ‘true’
--> Explanation: Boolean can only be assigned true or false literals
What is the output of this program?
class mainclass {
public static void main(String args[])
{
char a = 'A';
a++;
System.out.print((int)a);
}
}
a) 66
b) 67
c) 65
d) 64
--> ASCII value of ‘A’ is 65, on using ++ operator character value increments by one.
What is the output of this program?
class booloperators {
public static void main(String args[])
{
boolean var1 = true;
boolean var2 = false;
System.out.println((var2 & var2));
}
}
a) 0
b) 1
c) trued) false
--> boolean ‘&’ operator always returns true or false. var1 is defined true and var2 is defined false hence their ‘&’ operator result is false.
Which of these is an incorrect array declaration?
a) int arr[] = new int[5]
b) int [] arr = new int[5]
c) int arr[] = new int[5]d) int arr[] = int [5] new
--> Operator 'new' must be succeeded by array type and array size.
What will this code print?
int arr[] = new int [5];
System.out.print(arr);
a) 0
b) value stored in arr[0].
c) 00000d) Class name@ hashcode in hexadecimal form
--> If we trying to print any reference variable internally, toString() will be called which is implemented to return the String in following form:
classname@hashcode in hexadecimal form
Which of these is an incorrect Statement?
a) It is necessary to use new operator to initialize an array.
b) Array can be initialized using comma separated expressions surrounded by curly braces.
c) Array can be initialized when they are declared.
d) None of the mentioned
--> Array can be initialized using both new and comma separated expressions surrounded by curly braces example : int arr[5] = new int[5]; and int arr[] = { 0, 1, 2, 3, 4};
Which of these is necessary to specify at time of array initialization?
a) Row
b) Column
c) Both Row and Column
d) None of the mentioned
Which of these is necessary condition for automatic type conversion in Java?
a) The destination type is smaller than source type.
b) The destination type is larger than source type.
c) The destination type can be larger or smaller than source type.
d) None of the mentioned
What is the error in this code?
byte b = 50;
b = b * 50;
a) b can not contain value 100, limited by its range.
b) * operator has converted b * 50 into int, which can not be converted to byte without casting.
c) b can not contain value 50.
d) No error in this code
If an expression contains double, int, float, long, then whole expression will promoted into which of these data types?
a) long
b) int
c) double
d) float
--> If any operand is double the result of expression is double.
What is Truncation is Java?
a) Floating-point value assigned to an integer type.
b) Integer value assigned to floating type.
c) Floating-point value assigned to an Floating type.
d) Integer value assigned to floating type.
What is the output of this program?
class c {
public void main( String[] args )
{
System.out.println( "Hello" + args[0] );
} }
a) Hello c
b) Hello
c) Hello world
d) Runtime Error.
--> A runtime error will occur owning to the main method of the code fragment not being declared static.
Which of these keywords is used to refer to member of base class from a sub class?
a) upper
b) super
c) this
d) None of the mentioned
--> Whenever a subclass needs to refer to its immediate superclass, it can do so by use of the keyword super.
Which of these method of String class can be used to test to strings for equality?
a) isequal()
b) isequals()
c) equal()
d) equals()
Which of the following statements are incorrect?
a) String is a class.
b) Strings in java are mutable.
c) Every string is an object of class String.
d) Java defines a peer class of String, called StringBuffer, which allows string to be altered.
---> Strings in Java are immutable that is they can not be modified.
What is the output of this program?
class string_class {
public static void main(String args[])
{
String obj = "I LIKE JAVA";
System.out.println(obj.charAt(3));
} }
a) I
b) L
c) K
d) E
charAt() is a method of class String which gives the character specified by the index. obj.charAt(3) gives 4th character i:e I.
What is the output of this program?
class string_class {
public static void main(String args[])
{
String obj = "hello";
String obj1 = "world";
String obj2 = "hello";
System.out.println(obj.equals(obj1) + " " + obj.equals(obj2));
}
}
a) false false
b) true true
c) true false
d) false true
--> equals() is method of class String, it is used to check equality of two String objects, if they are equal, true is retuned else false.
Which of these is correct way of inheriting class A by class B?
a) class B + class A {}
b) class B inherits class A {}
c) class B extends A {}
d) class B extends class A {}
What is the output of this program?
class A {
public int i;
private int j;
}
class B extends A {
void display() {
super.j = super.i + 1;
System.out.println(super.i + " " + super.j);
}
}
class inheritance {
public static void main(String args[])
{
B obj = new B();
obj.i=1;
obj.j=2;
obj.display();
} }
a) 2 2
b) 3 3
c) Runtime Error
d) Compilation Error
--> class contains a private member variable j, this cannot be inherited by subclass B and does not have access to it.
Which of these is correct way of calling a constructor having no parameters, of superclass A by subclass B?
a) super(void);
b) superclass.();
c) super.A();
d) super();
Which of these method of Object class is used to obtain class of an object at run time?
a) get()
b) void getclass()
c) Class getclass()
d) None of the mentioned
Which of these keywords cannot be used for a class which has been declared final?
a) abstract
b) extends
c) abstract and extends
d) None of the mentioned
---> An abstract class is incomplete by itself and relies upon its subclasses to provide complete implementation. If we declare a class final then no class can inherit that class, an abstract class needs its subclasses hence both final and abstract cannot be used for a same class.
Which of these class relies upon its subclasses for complete implementation of its methods?
a) Object class
b) abstract class
c) ArrayList class
d) None of the mentioned
What is the output of this program?
class A {
int i;
int j;
A() {
i = 1;
j = 2;
}
}
class Output {
public static void main(String args[])
{
A obj1 = new A();
A obj2 = new A();
System.out.print(obj1.equals(obj2));
} }
a) false
b) true
c) 1
d) Compilation Error
---> obj1 and obj2 are two different objects. equals() is a method of Object class, Since Object class is superclass of every class it is available to every object.
Which of these statement is correct?
a) true and false are numeric values 1 and 0.
b) true and false are numeric values 0 and 1.
c) true is any non zero value and false is 0.
d) true and false are non numeric values.
---> true and false are keywords, they are non numeric values which do no relate to zero or non zero numbers. true and false are boolean values.
What is the value returned by function compareTo() if the invoking string is greater than the string compared?
a) zero
b) value less than zero
c) value greater than zero
d) None of the mentioned
Which of these method of class StringBuffer is used to get the length of sequence of characters?
a) length()
b) capacity()
c) Length()
d) Capacity()
Which of the following are incorrect form of StringBuffer class constructor?
a) StringBuffer()
b) StringBuffer(int size)
c) StringBuffer(String str)
d) StringBuffer(int size , String str)
What is the output of this program?
class output {
public static void main(String args[]) {
StringBuffer sb=new StringBuffer("Hello");
sb.replace(1,3,"Java");
System.out.println(sb);
} }
a) Hello java
b) Hellojava
c) HJavalo
d) Hjava
Which of these method of class String is used to extract more than one character at a time a String object?
a) getchars()
b) GetChars()
c) Getchars()
d) getChars()
In below code, what can directly access and change the value of the variable name?
package test;
class Target
{
public String name = "hello"; }
a) any class
b) only the Target class
c) any class in the test package
d) any class that extends Target
Which of these methods can be used to convert all characters in a String into a character array?
a) charAt()
b) both getChars() & charAt()
c) both toCharArray() & getChars()
d) All of the mentioned
---> charAt() return one character only not array of character.
Which of the following statements is correct?
a) Public method is accessible to all other classes in the hierarchy
b) Public method is accessible only to subclasses of its parent class
c) Public method can only be called by object of its class.
d) Public method can be accessed by calling object of the public class.
What is the output of this program?
class box {
int width;
int height;
int length;
}
class mainclass {
public static void main(String args[])
{
box obj = new box();
System.out.println(obj);
}
}
a) 0
b) 1
c) Runtime error
d) classname@hashcode in hexadecimal form
When we print object internally toString() will be called to return string into this format classname@hashcode in hexadecimal form.
-> output box@130671e
What is process of defining two or more methods within same class that have same name but different parameters declaration?
a) method overloading
b) method overriding
c) method hiding
d) None of the mentioned
Two or more methods can have same name as long as their parameters declaration is different, the methods are said to be overloaded and process is called method overloading. Method overloading is a way by which Java implements polymorphism
What is the process by which we can control what parts of a program can access the members of a class?
a) Polymorphism
b) Abstraction
c) Encapsulation
d) Recursion
Arrays in Java are implemented as?
a) class
b) object
c) variable
d) None of the mentioned
Which of the following statements are incorrect?
a) static methods can call other static methods only.
b) static methods must only access static data.
c) static methods can not refer to this or super in any way.
d) when object of class is declared, each object contains its own copy of static variables.
Which of these access specifiers can be used for an interface?
a) Public
b) Protected
c) private
d) All of the mentioned
--> Access specifier of interface is either public or no specifier. When no access specifier is used then default access specifier is used due to which interface is available only to other members of the package in which it is declared, when declared public it can be used by any code.
Which of these method is a rounding function of Math class?
a) max()
b) min()
c) abs()
d) All of the mentioned
What is the output of this program?
class Output {
public static void main(String args[]) {
double x = 2.0;
double y = 3.0;
double z = Math.pow( x, y );
System.out.print(z);
}
}
a) 2.0
b) 4.0
c) 8.0
d) 9.0
---> Math.pow(x, y) methods returns value of y to the power x, i:e x ^ y, 2.0 ^ 3.0 = 8.0.
If a class inheriting an abstract class does not define all of its function then it will be known as?
a) abstract
b) A simple class
c) Static class
d) None of the mentioned
--> Any subclass of an abstract class must either implement all of the abstract methods in the superclass or be itself declared abstract.
Which of these is not a correct statement?
a) Every class containing abstract method must be declared abstract.
b) Abstract class defines only the structure of the class not its implementation.
c) Abstract class can be initiated by new operator.
d) Abstract class can be inherited.
--> Abstract class cannot be directly initiated with new operator, Since abstract class does not contain any definition of implementation it is not possible to create an abstract object.
Which of these class is used to read characters and strings in Java from console?
a) BufferedReader
b) StringReader
c) BufferedStreamReader
d) InputStreamReader
Which of these class contains the methods print() & println()?
a) System
b) System.out
c) BUfferedOutputStream
d) PrintStream
Which class cannot be a subclass in java?
a) Abstract class
b) Parent class
c) Final class
d) None of the above
Which of the following can be operands of arithmetic operators?
a) Numeric
b) Boolean
c) Characters
d) Both Numeric & Characters
---> The operand of arithmetic operators can be any of numeric or character type, But not boolean.
With x = 0, which of the following are legal lines of Java code for changing the value of x to 1?
1. x++;
2. x = x + 1;
3. x += 1;
4. x =+ 1;
a) 1, 2 & 3
b) 1 & 4
c) 1, 2, 3 & 4
d) 3 & 2
Which of these have highest precedence?
a) ()
b) ++
c) *
d) >>
---> Order of precedence is (highest to lowest) a -> b -> c -> d
What should be expression1 evaluate to in using ternary operator as in this line?
expression1 ? expression2 : expression3
a) Integer
b) Floating – point numbers
c) Boolean
d) None of the mentioned
---> The controlling condition of ternary operator must evaluate to boolean.
Which of these statement is incorrect?
a) switch statement is more efficient than a set of nested ifs.
b) two case constants in the same switch can have identical values.
c) switch statement can only test for equality, whereas if statement can evaluate any type of boolean expression.
d) it is possible to create a nested switch statements.
b) two case constants in the same switch can have identical values.
---> No two case constants in the same switch can have identical values.
What is the stored in the object obj in following lines of code?
box obj;
a) Memory address of allocated memory of object.
b) NULL
c) Any arbitrary pointer
d) Garbage
---> Memory is allocated to an object using new operator. box obj; just declares a reference to object, no memory is allocated to it hence it points to NULL.
Which of the following statements are incorrect?
a) public members of class can be accessed by any code in the program.
b) private members of class can only be accessed by other members of the class.
c) private members of class can be inherited by a sub class, and become protected members in sub class.
d) protected members of a class can be inherited by a sub class, and become private members of the sub class.
---> private members of a class cannot be inherited by a sub class.
At line number 2 below, choose 3 valid data-type attributes/qualifiers among “final, static, native, public, private, abstract, protected”
public interface Status
{
/* insert qualifier here */ int MY_VALUE = 10;
}
a) final, native, private
b) final, static, protected
c) final, private, abstract
d) final, static, public
--> Every interface variable is implicitly public static and final.
The question on the page originate from the summary of the following study material:
- A unique study and practice tool
- Never study anything twice again
- Get the grades you hope for
- 100% sure, 100% understanding
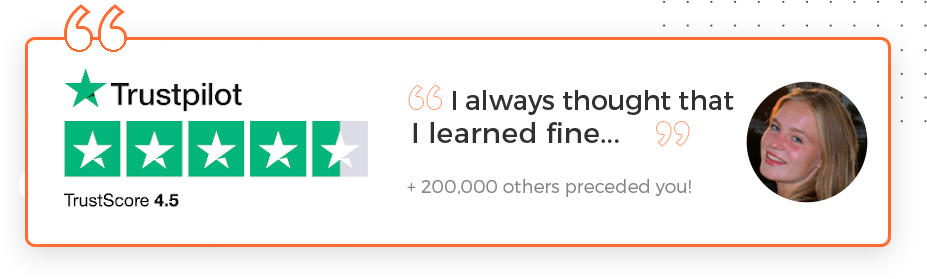