Programming psychopy
19 important questions on Programming psychopy
How do you present a participant a window with text?
msg = visual.TextStim(win, text = "hello world", colorSpace = "rgb255", color = (255,255,255))
#prepare text message on win
msg.draw() #actually place it in the window win
win.flip() #show it to the participant
core.wait(1) #window is presented for 1 second
#win.close() #window disappears, win close is only used at the end of experiment can leave it out in between
What is a very important aspect in your designing phase?
type: win = visual.Window(fullscr = False)
How can you adjust the colour of your window and other items?
values for input go from 0 to 255, so color = (255,0,0) will give a full red screen
important!
always set ColorSpace = "rgb255"
otherwise the code will not be recognized
in case of an itemcolor for example a line: lineColorSpace = "rgb255"
- Higher grades + faster learning
- Never study anything twice
- 100% sure, 100% understanding
How can you draw a rectangle on the screen?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- from psychopy import core, visual
- win = visual.Window(units='pix')
- rectangle = visual.Rect(win, units = 'pix',size = (75,44), lineWidth = 3, lineColorSpace = "rgb255", lineColor = (255,0,255), fillColorSpace = "rgb255", fillColor = (255,128,0)) # the object rectangle is now made with specific properties
- rectangle.pos = (-100,50) #define rectangle position
- rectangle.draw() # rectangle is placed on the window
- win.flip() #window is presented
- core.wait(2) # two second presentation
- #win.close() #window is closed
How does the positioning function work?
- The function is item_name.pos = (x,y)
- First index is the x-position, second index the y-position.
- -400: 400 to the left of center; 200: 200 to the top of the center.
- So X goes from left (-) to right (+); Y goes from bottom (-) to top (+).
How can you present a circle on the screen?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- from psychopy import core, visual
- win = visual.Window(fullscr=False, colorSpace='rgb255', color=[0,0,0], units='pix')
- circle = visual.Circle(win, units = 'pix', radius = 75, lineWidth = 3, lineColorSpace = "rgb255", lineColor = (0,255,0), fillColor = None) #radius of the circle is now 300 pixels, thus diameter of 600
- circle.pos = (100,-50) # x: - is naar links, y: - is naar beneden. Dus linksboven = -X, Y
- circle.draw()
- win.flip()
- core.wait(2)
- win.close()
How can you show a picture on the screen?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- from psychopy import core, visual
- win = visual.Window(fullscr=False, colorSpace='rgb255', color=[0,0,0], units='pix')
- pict = visual.ImageStim(win, image='Cat1.jpg', units = 'pix', size = (80,80))
- pict.pos = (0,100)
- pict.draw()
- win.flip()
- core.wait(2)
- win.close()
How do i present a video?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- from psychopy import core, visual
- from psychopy import sound, gui, data, event, logging, clock, colors
- win = visual.Window(fullscr=False, colorSpace='rgb255', color=[0,0,0], units='pix')
- clip = visual.MovieStim3(win, noAudio = False, filename='Catvideo1.mp4’, size = (80,80), loop=True)
- clip.pos = (0,100)
- trialClock = core.Clock() #creates a clock time
- timeold = trialClock.getTime() # to get the initial time
- stoppen = 0
- while stoppen == 0:
- clip.setAutoDraw(True) #next frame will automatically be selected
- win.flip() #frame shown
- timenow = trialClock.getTime() #time updated
- timediff = timenow - timeold #time difference calculated
- if timediff>4 :
- stoppen = 1
- win.close()
How can you create a gui: a general user interface where you can have participants fill in information before an experiment starts?
- expInfo = {'participant': 'yp', 'session': '001', 'typeD': '1'} #specify library of items which will be in the gui
- "participant": "yp" #by default is yp unless specified otherwise
- dlg = gui.DlgFromDict(dictionary=expInfo, title='ExampleGui')
- #creates the actual gui
- if dlg.OK == False:
- core.quit()
Note: you do not have to define a Window, use draw() or flip() or anything like it. If you run the code where you define a GUI, then the GUI immediately appears on the screen
How do you make a gui script?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- from psychopy import core, visual
- from psychopy import sound, gui, data, event, logging, clock, colors
- expInfo = {'participant': 'yp', 'session': '001', 'typeD': '1'}
- dlg = gui.DlgFromDict(dictionary=expInfo, title='ExampleGui')
- if dlg.OK == False:
- core.quit() # user pressed cancel
- pp = expInfo['participant']
- sessnr = expInfo['session']
- distractorType = int(expInfo['typeD'])
what is filled in in the gui is overwritten in expinfo and can thus be accessed by indexing expinfo
!!! Keep in mind to access parts of the dictionary, you can only index them by name, not by position.
expInfo['participant'] will work,
expInfo[0] will not work
How do you put the information from the gui into a file?
- thisExp = data.ExperimentHandler(dataFileName=filename)
- #The name of the datafile is filename. This should be a string that we have to define before using it!
- thisExp.addData('Name', pp)
- #Add data to the file. Specifically: under the header ‘Name’
- thisExp.nextEntry()
- #Go to the next row in the excel file.
- _
- *information*
- _
- thisExp.saveAsWideText(filename+'.csv', delim='auto’)
- #This command appears at the end, after you have saved all the data you want to the thisExp.
- thisExp.abort()
- #This command needs to be inserted after you save the data file to prevent double saving after the experiment stops.
How can you input keyboard responses?
- Kb = keyboard.Keyboard() #this opens a keyboard called kb
- # important argument: waitForStart = True/False
- False by default: now records from keyboard all the time if set to True only starts recording after kb.start() and stops after kb.stop()
- keys = kb.getKeys() #makes item key which is a list amongst which the input is saved
- keys = kb.waitKeys() #makes item key which is a list amongst which the input is saved
- wait.Keys() has a built in while loop which waits for a response
- arguments for both:
- keyList = () #provide a list of which buttons are recorded, if left empty all buttons will be recorded
- waitRelease = T/F #key only recorded upon release
- clear = T/F #after recording clears working space.
keys[0]link6
apparently the list keys contains more than just the response. This is how you find which button has been pressed.
How can you present stimuli with positions which vary with a forloop?
x_positions = [-150,-150,150,150]
y_positions = [150,-150,150,-150]
circle = visual.Circle(win, units = 'pix', radius = 75, lineWidth = 3, lineColorSpace = "rgb255", lineColor = (0,255,0), fillColor = None) #radius of the circle is now 300 pixels, thus diameter of 600
rectangle2 = visual.Rect(win, units = 'pix',size = (75,44), lineWidth = 3, lineColorSpace = "rgb255", lineColor = (255,0,255), fillColorSpace = "rgb255", fillColor = None)
for i in range(0,4):
if i == 3:
rectangle2.pos = (x_positions[i], y_positions[i])
rectangle2.draw()
else:
circle.pos = (x_positions[i], y_positions[i])
circle.draw()
win.flip()
core.wait(2)
How can you present a message to a participant untill he presses a key?
- kb = keyboard.Keyboard() #this opens a keyboard called kb
- msg = ""
- msg.draw() #actually place it in the window win
- win.flip() #show it to the participant
- start = False
- while start == False:
- startkey = kb.waitKeys(keyList = ("z", "m"))
- if startkey[0]link3 == "z" or "m":
- start = True
- win.flip()
make sure win.flip is at the bottom of the while loop, only then it works.
How can you show a video until a key is pressed?
win = visual.Window(fullscr=False, colorSpace='rgb255', color=[0,0,0], units='pix')
clip = visual.MovieStim3(win, noAudio = False, filename="catvideo.mp4", size = (80,80), loop=True)
ingedrukt = 0
pressed = False
kb.start()
while ingedrukt == 0:
clip.setAutoDraw(True)
win.flip()
theseKeys = kb.getKeys(keyList=['z', 'm'], waitRelease=False)
if theseKeys:
kb.stop()
ingedrukt = 1
How can you make a fully random position decider?
random.randint(0,x)
#x = amount of possible positions - 1, value x is taken int calculation
# for two possible positions: random.randint(0, 1)
How can you make a controlled random position decider, so you control still how often everything occurs but just the order randomized?
position_list = [0,0,0,1,1,1]
random.shuffle(position_list)
#now loop through the list to select the positions.
How can you present a stimulus until the participant presses a screen?
from psychopy import core, gui, visual
from psychopy.hardware import keyboard
stimulus_position = random.randint(0, 1)
circle.draw()
if stimulus_position == 0:
stimulus.pos = (-200,0)
else:
stimulus.pos = (200,0)
stimulus.draw()
win.flip()
stop = False
while stop == False:
startkey = kb.waitKeys(keyList = ("z", "m"))
if startkey[0]link8 == "z" or "m":
stop = True win.flip()
What is a neat way of ordering your code for an experiment?
- #!/usr/bin/env python
- # -*- coding: utf-8 -*-
- imports
- define functions
- define all variables
- then make your code
- exit code: close win, close file etc.
The question on the page originate from the summary of the following study material:
- A unique study and practice tool
- Never study anything twice again
- Get the grades you hope for
- 100% sure, 100% understanding
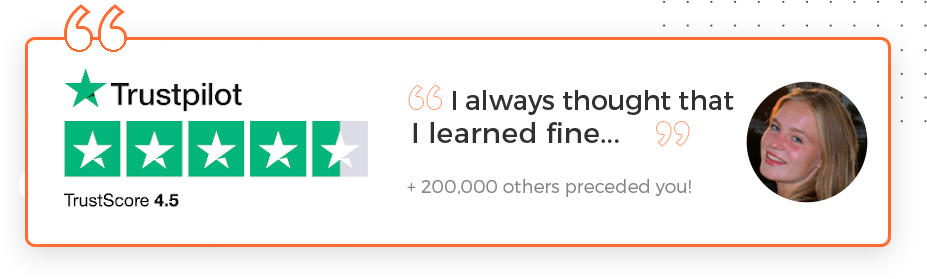